
- PHP - Home
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP cURL curl_exec() Function
The PHP Client URL curl_exec() function is used to execute the given cURL session. This function must be called after you have initialized a cURL session and set all of its settings.
This function may return the Boolean false, but it can also return a non-Boolean value that evaluates to false. Use the === operator to test this function's return value.
Syntax
Below is the syntax of the PHP cURL curl_exec() function −
mixed curl_exec(resource $ch)
Parameters
This function accepts $ch parameter which is the cURL handle resource returned by curl_init().
Return Value
The curl_exec() function returns the fetched data as a string on success, or FALSE if an error occurs.
But if the CURLOPT_RETURNTRANSFER option is enabled, the result will be returned on success and FALSE on failure.
PHP Version
First introduced in core PHP 4.0.2, the curl_exec() function continues to function easily in PHP 5, PHP 7, and PHP 8.
Example 1
First we will show you the basic example of the PHP cURL curl_exec() function to execute the given URL to the browser.
<?php // Create a new cURL resource $ch = curl_init(); // set the URL and other options curl_setopt($ch, CURLOPT_URL, "http://www.example.com/"); curl_setopt($ch, CURLOPT_HEADER, 0); // Get URL and send it to the browser curl_exec($ch); // Close the cURL resource to free up the resources curl_close($ch);
Output
The content will be displayed of the given URL −
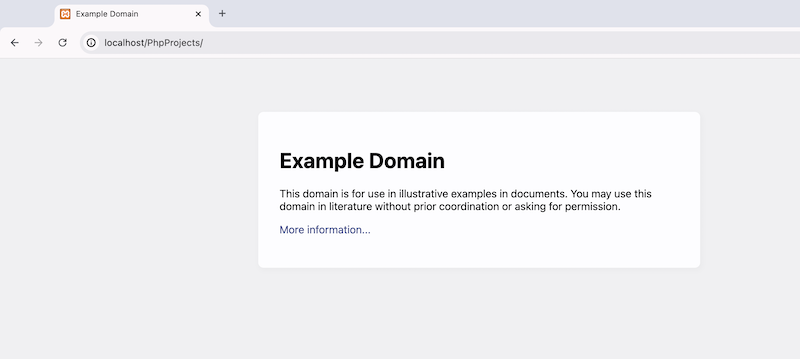
Example 2
In the below PHP code we will try to use the curl_exec() function and execute the request to fetch data from a URL.
<?php // Initialize a cURL session $ch = curl_init('https://tutorialspoint.com/api/data'); // Set cURL options if needed curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the request $response = curl_exec($ch); // Check for errors if($response === false) { echo 'cURL Error: ' . curl_error($ch); } // Close cURL session curl_close($ch); echo $response; ?>
Output
This will show the content of the given URL −

Example 3
Now we will use the curl_exec() function and call a rest api to get the repos of the github user.
<?php // GitHub username $username = 'abc'; // Replace with the GitHub username you want to fetch repositories for // API endpoint URL $url = "https://api.github.com/users/{$username}/repos"; // Initialize cURL session $ch = curl_init($url); // Set options for sending a GET request curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, [ 'Accept: application/json', 'User-Agent: Your-App-Name' // Replace with your own User-Agent header ]); // Execute the request $response = curl_exec($ch); // Check for errors if($response === false) { echo 'cURL Error: ' . curl_error($ch); } else { // Decode JSON response $data = json_decode($response, true); // Check if decoding was successful if($data === null) { echo 'Error decoding JSON'; } else { // Process API response data echo 'GitHub Repositories:'; foreach ($data as $repo) { echo '<br>'; echo 'Name: ' . $repo['name'] ; echo '<br>'; echo 'URL: ' . $repo['html_url'] . '<br>'; echo '--------------------------'; } } } // Close cURL session curl_close($ch); ?>
Output
This will create the below output −
GitHub Repositories: Name: admin-dashboard URL: https://github.com/abc/admin-dashboard -------------------------- Name: b2brain-application URL: https://github.com/abc/b2brain-application -------------------------- Name: redux-github-repo URL: https://github.com/abc/redux-github-repo -------------------------- Name: responsive-portfolio URL: https://github.com/abc/responsive-portfolio -------------------------- Name: To-do-list URL: https://github.com/abc/To-do-list --------------------------
Important Note
When calling curl_exec() in PHP, response status codes like 404 (Not Found) are not considered failures. You can look for these status codes with curl_getinfo().