
- PHP - Home
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP cURL curl_pause() Function
The PHP cURL curl_pause() function is used to pause or unpause a connection or cURL session. To pause a session at the time of a transfer that is happening in either the read, write, or both directions, this function can be used from a callback that has been registered with curl_setopt().
Syntax
Below is the syntax of the PHP cURL curl_pause() function −
int curl_pause (resource $ch, int $flags)
Parameters
Below are the parameters of the curl_pause() function −
$ch − It is the cURL handle returned by curl_init().
$flags − It is an integer CURLPAUSE_* constants that shows which connections should be suspended and which ones should not.
CURLPAUSE_* Constants
The available constants are as follows:
- CURLPAUSE_RECV: Pause receiving data.
- CURLPAUSE_RECV_CONT: Unpause receiving data.
- CURLPAUSE_SEND: Pause sending data.
- CURLPAUSE_SEND_CONT: Unpause sending data.
- CURLPAUSE_ALL: Pause both receiving and sending data.
- CURLPAUSE_CONT: Unpause both receiving and sending data.
Return Value
The curl_pause() function returns 0 (CURLE_OK) on success and cURL error code on failure.
PHP Version
First introduced in core PHP 5.5.0, the curl_pause() function continues to function easily in PHP 7, and PHP 8.
Example 1
Here is the basic example of the PHP cURL curl_pause() function to pause and unpause the receiving data. And we have used CURLPAUSE_RECV constant to pause the receiving data.
<?php $ch = curl_init("http://abc123.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Start the transfer curl_exec($ch); // Pause receiving data curl_pause($ch, CURLPAUSE_RECV); // Pause receiving data curl_pause($ch, CURLPAUSE_RECV); echo "The curl_pause() function has paused receiving data!\n"; // Unpause receiving data curl_pause($ch, CURLPAUSE_RECV_CONT); echo "The curl_pause() function has unpaused receiving data!"; curl_close($ch); ?>
Output
Here is the outcome of the following code −
The curl_pause() function has paused receiving data! The curl_pause() function has unpaused receiving data!
Example 2
In the below PHP code we will use the curl_pause() function with CURLPAUSE_ALL constant to pause both the sending and receiving data.
<?php // Create a cURL handle $ch = curl_init("http://example.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Start the transfer $output = curl_exec($ch); // Pause both sending and receiving data curl_pause($ch, CURLPAUSE_ALL); // Simulate some processing or delay sleep(2); // Unpause both sending and receiving data curl_pause($ch, CURLPAUSE_CONT); curl_close($ch); // Print the output echo $output; ?>
Output
It will display the Content of the Provided URL −
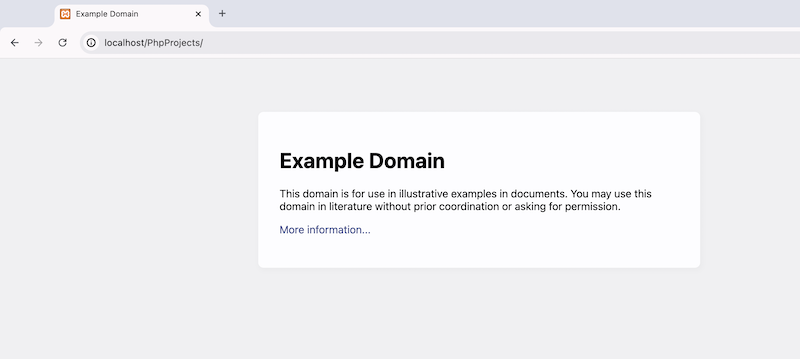
Example 3
Now we will use the curl_pause() function with CURLPAUSE_SEND constant to pause only sending data for 4 seconds.
<?php // Create the cURL handle $ch = curl_init("http://abc123.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Start the transfer and store the result $output = curl_exec($ch); // Pause sending data curl_pause($ch, CURLPAUSE_SEND); // Simulate some processing or delay sleep(4); // Unpause sending data curl_pause($ch, CURLPAUSE_SEND_CONT); curl_close($ch); // Print the output echo $output; ?>
Output
Here the content of the provided URL will be displayed −
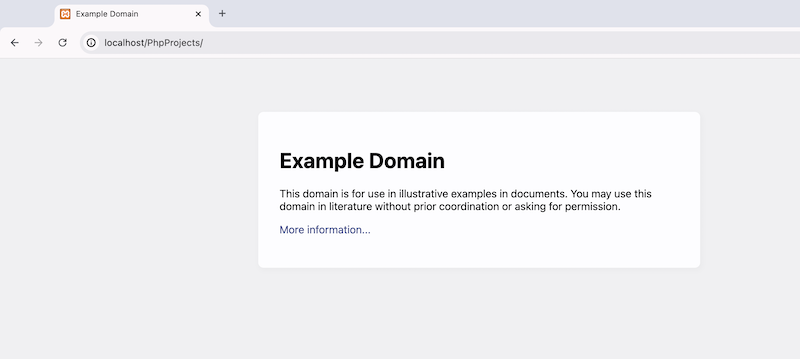
Example 4
Now use curl_pause() function with CURLPAUSE_RECV and CURLPAUSE_RECV_CONT to pause and unpause the receiving data and also we will provide conditional logic here.
<?php // Create the cURL handle $ch = curl_init("http://example.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Start the transfer and store the result $output = curl_exec($ch); // Check some condition before pausing if (strlen($output) > 1000) { // Pause receiving data if the output length is greater than 1000 characters curl_pause($ch, CURLPAUSE_RECV); // Simulate some processing or delay sleep(5); // Unpause receiving data curl_pause($ch, CURLPAUSE_RECV_CONT); } curl_close($ch); // Print the output echo $output; ?>
Output
It will also display the content of the URL after pausing for 5 seconds −
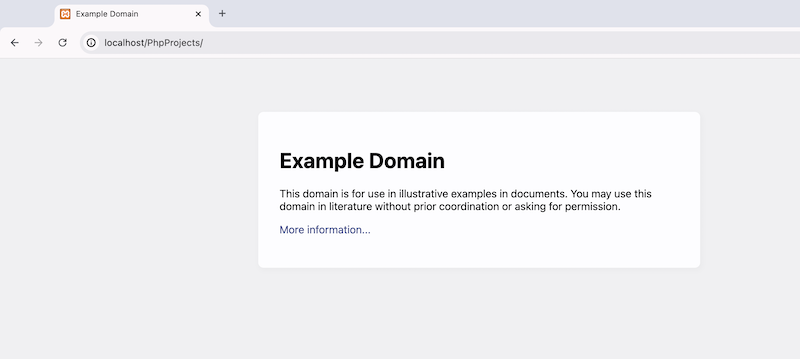