
- PHP - Home
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP cURL curl_reset() Function
The PHP cURL curl_reset() function is used to reset all the settings on the given cURL handle to their initial settings. This means that anything you have set up for a cURL session will be removed and you can start a new with the default settings.
Syntax
Below is the syntax of the PHP cURL curl_reset() function −
void curl_reset($ch)
Parameters
This function accepts $ch parameter which is the cURL session handle that you want to reset.
Return Value
The curl_reset() function only performs action and does not return any value.
PHP Version
This function was first introduced as part of core PHP 5.5.0 and work well with the PHP 7, PHP 8.
Example 1
First we will show you the basic example of the PHP cURL curl_reset() function to reset the settings on the given URL.
<?php // Start a curl handle $ch = curl_init(); // Set CURLOPT_USERAGENT option curl_setopt($ch, CURLOPT_USERAGENT, "My test user-agent"); // Reset all previously set options curl_reset($ch); // Send HTTP request curl_setopt($ch, CURLOPT_URL, 'http://abc123.com/'); curl_exec($ch); // Close the handle curl_close($ch); ?>
Output
This code will show the content of the given URL −
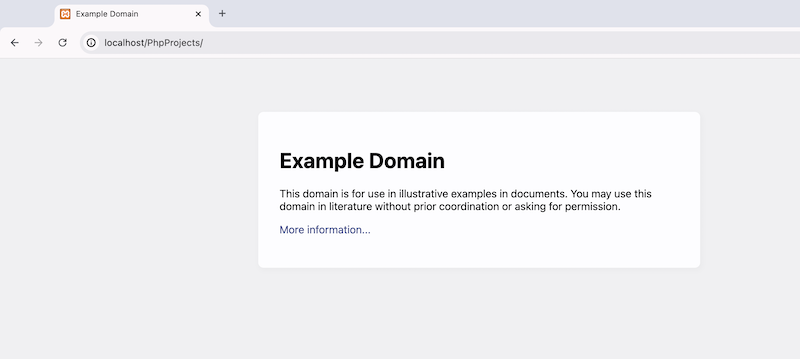
Example 2
In the below PHP code we will use the curl_reset() function and reset the cURL session and set new options for the cURL session.
<?php // Create a cURL session $ch = curl_init(); // Set some options for the cURL session curl_setopt($ch, CURLOPT_URL, "http://example.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the cURL session $response = curl_exec($ch); // Reset the cURL session handle curl_reset($ch); // Now you can set new options for the cURL session curl_setopt($ch, CURLOPT_URL, "http://anotherexample.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the cURL session again $response = curl_exec($ch); echo "It has reset all the settings on the given cURL handle!"; // Close the cURL session curl_close($ch); ?>
Output
Here is the output of the above code −
It has reset all the settings on the given cURL handle!
Example 3
In the below code, using the curl_reset() function we will reuse a cURL handle for multiple GET requests.
<?php // Create a cURL session $ch = curl_init(); // Set options for the first request curl_setopt($ch, CURLOPT_URL, "http://example.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the first request $res1 = curl_exec($ch); echo "Response from 1st request: " . $res1 . "\n"; // Reset the cURL session handle curl_reset($ch); // Set options for the second request curl_setopt($ch, CURLOPT_URL, "http://abc123.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the second request $res2 = curl_exec($ch); echo "Response from 2nd request: " . $res2 . "\n"; // Close the cURL session curl_close($ch); ?>
Output
It will show the content of both the URLs provided −

Example 4
In the following example, we are using the curl_reset() function to reuse a cURL handle for both the GET and POST requests.
<?php // Create a cURL session $ch = curl_init(); // Set options for the GET request curl_setopt($ch, CURLOPT_URL, "http://example.com"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the GET request $res1 = curl_exec($ch); echo "Response from GET request: " . $res1 . "\n"; // Reset the session handle curl_reset($ch); // Set options for the POST request curl_setopt($ch, CURLOPT_URL, "http://example.org"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, "param1=value1¶m2=value2"); // Execute the POST request $res2 = curl_exec($ch); echo "Response from POST request: " . $res2 . "\n"; // Close the session curl_close($ch); ?>
Output
Here is the content from both the URLs will be displayed −
