
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Control Flow
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - Emitting Code
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium - Double Click
- Selenium - Right Click
- HTML Report in Python
- Handling Edit Boxes
- Selenium - Single Elements
- Selenium - Multiple Elements
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Pen Events
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - ThreadGuard
- Selenium - Errors & Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium - DevTools
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github Tutorial
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - IE Driver
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
Selenium Webdriver - Select Support
Selenium Webdriver can be used to select options in a dropdown boxes on a web page with the help of the Select class. There can be two types of dropdown on a web page - single select(allows selection one option) and multiple select(allows selection more than one option).
A dropdown is identified by the tagname known as the select. Besides, each of its options have the tagname called the option. Moreover, a multiple select dropdown contains an attribute multiple.
Identification of Dropdown in HTML
Right click on the webpage, and then click on the Inspect button in the Chrome browser. Then, the corresponding HTML code for the whole page would be visible. For investigating the dropdown on the web page, we click on the left upward arrow, available to the top of the visible HTML code as highlighted below.

Once, clicked and then pointed the arrow to the dropdown beside the text Select One, its HTML code was visible, reflecting the select tagname(enclosed in <>), and its options within the option tagname.
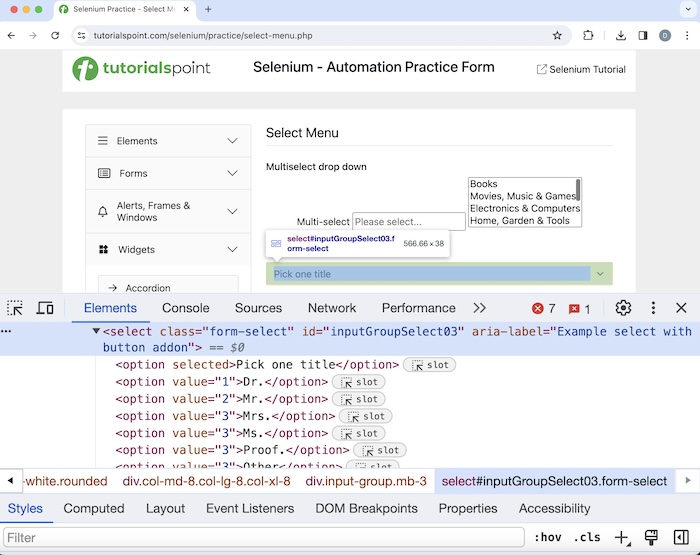
Please note one of the options Pick one title has the attribute selected, meaning this is selected by default, even before any option is selected. Moreover, if a dropdown list contains an attribute value as disabled, that option can not be selected.
Basic Select Methods
There are multiple methods available in the Select class of Selenium webdriver which helps us to handle both the multiple, and single select dropdowns.
Example 1 - Single Select Dropdown
Let us take an example of the below page, where we would access the dropdown below the text Select One, select the value Others and perform some validations with the methods discussed above.

Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class SelectDropdowns { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://www.tutorialspoint.com/selenium/practice/select-menu.php"); // identify dropdown then select its options by index WebElement dropdown = driver.findElement (By.xpath("//*[@id='inputGroupSelect03']")); Select select = new Select(dropdown); // get option selected by default WebElement o = select.getFirstSelectedOption(); System.out.println("Option selected by default: " + o.getText()); // select an option by index select.selectByIndex(6); // get selected option List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Option is: " + opt.getText()); } // get all options of dropdown List<WebElement> options =select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // check if multiselect dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for checking is: "+ b); // quitting browser driver.quit(); } }
Output
Option selected by default: Pick one title Selected Option is: Other Options are: Pick one title Options are: Dr. Options are: Mr. Options are: Mrs. Options are: Ms. Options are: Proof. Options are: Other Boolean value for checking is: false Process finished with exit code 0
In the above example, we had obtained the selected option in the dropdown with the message in the console - Selected Option is: Other. Then obtain all the options of the dropdown with the message in the console - Options are: Pick one title, Options are: Dr., Options are: Africa, Options are: Mr., Options are: Mrs., Options are: Ms, Options are: Proof., and Options are: Other.
We had also validated that the dropdown did not have multiple selection options with the message in the console - Boolean value for checking is: false. We had also retrieved the option selected by default in the dropdown with the message in the console - Option selected by default: Pick one title.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2 - Multi Select Dropdown
Let us take another example of the below page, where we would access the multi-select dropdown beside the text Multi Select drop down, select the values Electronics & Computers and Sports & Outdoors, and perform some validations with the methods discussed above.

Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class SelectMultipleDropdowns { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://www.tutorialspoint.com/selenium/practice/select-menu.php"); // identify multiple dropdown WebElement dropdown = driver.findElement (By.xpath("//*[@id='demo-multiple-select']")); // object of Select class Select select = new Select(dropdown); // gets options of dropdown in list List<WebElement> options = select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // return true if multi-select dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for multiple dropdown: "+ b); // select item by value select.selectByValue("3"); // select item by index select.selectByIndex(7); // get all selected options of dropdown in list List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Options are: " + opt.getText()); } // get first selected option in dropdown WebElement f = select.getFirstSelectedOption(); System.out.println("First selected option is: "+ f.getText()); // deselect option by index select.deselectByIndex(7); // deselect all selected items select.deselectAll(); // get all selected options of dropdown after deselected List<WebElement> delectedOptions = select.getAllSelectedOptions(); System.out.println("No. options selected: " + delectedOptions.size()); // Closing browser driver.quit(); } }
Output
Options are: Books Options are: Movies, Music & Games Options are: Electronics & Computers Options are: Home, Garden & Tools Options are: Health & Beauty Options are: Toys, Kids & Baby Options are: Clothing & Jewelry Options are: Sports & Outdoors Boolean value for multiple dropdown: true Selected Options are: Electronics & Computers Selected Options are: Sports & Outdoors First selected option is: Electronics & Computers No. options selected: 0 Process finished with exit code 0
In the above example, we had obtained all the options of the dropdown with the message in the console - Options are: Books, Options are: Movies, Music & Games, Options are: Home, Garden & Tools, Options are: Health & Beauty, Options are: Toys, Kids & Baby, Options are: Clothing & Jewelry, Options are: Sports & Outdoors.
We had also validated that the dropdown had multiple selection options with the message in the console - Boolean value for checking is: true. We had retrieved the selected options in the dropdown with the message in the console - Selected Options are: Electronics & Computers, Selected Options are: Sports & Outdoors.
We had also obtained the first selected option with the message in the console - First selected option is: Electronics & Computers. Finally, we deselected all the selected options in the dropdown, and hence obtained the message in the console - No. options selected: 0.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
This concludes our comprehensive take on the tutorial on Selenium Webdriver - Select Support. Weve started with describing identification of Dropdown in HTML, basic select methods, and examples to illustrate how to handle single and multiple select dropdowns in Selenium Webdriver.
This equips you with in-depth knowledge of the Selenium Webdriver - Select Support. It is wise to keep practicing what youve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.