
- Selenium - Home
- Selenium - Overview
- Selenium - Components
- Selenium - Automation Testing
- Selenium - Environment Setup
- Selenium - Remote Control
- Selenium - IDE Introduction
- Selenium - Features
- Selenium - Limitations
- Selenium - Installation
- Selenium - Creating Tests
- Selenium - Creating Script
- Selenium - Control Flow
- Selenium - Store Variables
- Selenium - Alerts & Popups
- Selenium - Selenese Commands
- Selenium - Actions Commands
- Selenium - Accessors Commands
- Selenium - Assertions Commands
- Selenium - Assert/Verify Methods
- Selenium - Locating Strategies
- Selenium - Script Debugging
- Selenium - Verification Points
- Selenium - Pattern Matching
- Selenium - JSON Data File
- Selenium - Browser Execution
- Selenium - User Extensions
- Selenium - Code Export
- Selenium - Emitting Code
- Selenium - JavaScript Functions
- Selenium - Plugins
- Selenium WebDriver Tutorial
- Selenium - Introduction
- Selenium WebDriver vs RC
- Selenium - Installation
- Selenium - First Test Script
- Selenium - Driver Sessions
- Selenium - Browser Options
- Selenium - Chrome Options
- Selenium - Edge Options
- Selenium - Firefox Options
- Selenium - Safari Options
- Selenium - Double Click
- Selenium - Right Click
- HTML Report in Python
- Handling Edit Boxes
- Selenium - Single Elements
- Selenium - Multiple Elements
- Selenium Web Elements
- Selenium - File Upload
- Selenium - Locator Strategies
- Selenium - Relative Locators
- Selenium - Finders
- Selenium - Find All Links
- Selenium - User Interactions
- Selenium - WebElement Commands
- Selenium - Browser Interactions
- Selenium - Browser Commands
- Selenium - Browser Navigation
- Selenium - Alerts & Popups
- Selenium - Handling Forms
- Selenium - Windows and Tabs
- Selenium - Handling Links
- Selenium - Input Boxes
- Selenium - Radio Button
- Selenium - Checkboxes
- Selenium - Dropdown Box
- Selenium - Handling IFrames
- Selenium - Handling Cookies
- Selenium - Date Time Picker
- Selenium - Dynamic Web Tables
- Selenium - Actions Class
- Selenium - Action Class
- Selenium - Keyboard Events
- Selenium - Key Up/Down
- Selenium - Copy and Paste
- Selenium - Handle Special Keys
- Selenium - Mouse Events
- Selenium - Drag and Drop
- Selenium - Pen Events
- Selenium - Scroll Operations
- Selenium - Waiting Strategies
- Selenium - Explicit/Implicit Wait
- Selenium - Support Features
- Selenium - Multi Select
- Selenium - Wait Support
- Selenium - Select Support
- Selenium - Color Support
- Selenium - ThreadGuard
- Selenium - Errors & Logging
- Selenium - Exception Handling
- Selenium - Miscellaneous
- Selenium - Handling Ajax Calls
- Selenium - JSON Data File
- Selenium - CSV Data File
- Selenium - Excel Data File
- Selenium - Cross Browser Testing
- Selenium - Multi Browser Testing
- Selenium - Multi Windows Testing
- Selenium - JavaScript Executor
- Selenium - Headless Execution
- Selenium - Capture Screenshots
- Selenium - Capture Videos
- Selenium - Page Object Model
- Selenium - Page Factory
- Selenium - Record & Playback
- Selenium - Frameworks
- Selenium - Browsing Context
- Selenium - DevTools
- Selenium Grid Tutorial
- Selenium - Overview
- Selenium - Architecture
- Selenium - Components
- Selenium - Configuration
- Selenium - Create Test Script
- Selenium - Test Execution
- Selenium - Endpoints
- Selenium - Customizing a Node
- Selenium Reporting Tools
- Selenium - Reporting Tools
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & other Technologies
- Selenium - Java Tutorial
- Selenium - Python Tutorial
- Selenium - C# Tutorial
- Selenium - Javascript Tutorial
- Selenium - Kotlin Tutorial
- Selenium - Ruby Tutorial
- Selenium - Maven & Jenkins
- Selenium - Database Testing
- Selenium - LogExpert Logging
- Selenium - Log4j Logging
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash Testing
- Selenium - Apache Ant
- Selenium - Github Tutorial
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium Miscellaneous Concepts
- Selenium - IE Driver
- Selenium - Automation Frameworks
- Selenium - Keyword Driven Framework
- Selenium - Data Driven Framework
- Selenium - Hybrid Driven Framework
- Selenium - SSL Certificate Error
- Selenium - Alternatives
Selenium WebDriver - WebElement Commands
Selenium Webdriver can be used to extract multiple information about a particular element on a web page. For example, we can validate if an element is available/unavailable, enabled/disabled or selected/unselected on a web page.
Basic WebElement Commands in Selenium Webdriver
- To verify if an element is available on a web page, we can take the help of the isDisplayed() method. If the element is available, isDisplayed() would return true, else false.
- To verify if an element is selected on a web page, we can take the help of the isSelected() method. If the element is selected, isSelected() would return true, else false.
- To verify if an element is enabled on a web page, we can take the help of the isEnabled() method. If the element is enabled, isEnabled() would return true, else false.
- To get the tag name of an element, we can take the help of the getTagName() method.
- To get the x, and y coordinates of an element, we can take the help of the getRect() method.
- To get the background-color, or color of an element, we can take the help of the getCssValue() method.
- To fetch runtime value of the element associated with DOM we can take the help of the getAttribute() method and pass value as a parameter to that method.
- To get the text of an element, we can take the help of the getText() method.
Identify Radio Buttons in HTML
Right click on the webpage, and then click on the Inspect button in the Chrome browser. For inspecting a radio button on a page, click on the left upward arrow, available at the top of the visible HTML code as highlighted below.

Once we had clicked and pointed the arrow to the radio button (highlighted in the below image), its HTML code was seen, reflecting both the input tagname and value of type attribute as radio.

Example 1
Let us take an example of the above page, where we would click one of the radio buttons with the help of the click() method. Then we would verify if the radio button is available, enabled and selected using the above discussed methods.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ValidatingRadioButtons { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will click radio button driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // identify radio button then click WebElement radiobtn = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/div[1]/input")); radiobtn.click(); // verify if radio button is selected boolean result = radiobtn.isSelected(); System.out.println("Checking if a radio button is selected: " + result); // verify if radio button is displayed boolean result1 = radiobtn.isDisplayed(); System.out.println("Checking if a radio button is displayed: " + result1); // verify if radio button is enabled boolean result2 = radiobtn.isEnabled(); System.out.println("Checking if a radio button is enabled: " + result2); // identify another radio button WebElement radiobtn1 = driver. findElement(By.xpath("/html/body/main/div/div/div[2]/form/div[5]/input")); // verify if radio button is disabled boolean result3 = radiobtn1.isEnabled(); System.out.println("Checking if the other radio button is disabled: " + result3); // verify if radio button is unselected boolean result4 = radiobtn1.isEnabled(); System.out.println("Checking if the other radio button is unselected: " + result4); // Closing browser driver.quit(); } }
Output
Checking if a radio button is selected: true Checking if a radio button is displayed: true Checking if a radio button is enabled: true Checking if the other radio button is disabled: false Checking if the other radio button is unselected: false Process finished with exit code 0
In the above example, we had first clicked on a radio button, and then verified if the radio button was available, selected, and enabled in the console with the message - Checking if a radio button is selected: true, Checking if a radio button is displayed: true, Checking if a radio button is enabled: true.
Then we had validated for another radio button if it is disabled and unselected with the message in the console - Checking if the other radio button is disabled: false, and Checking if the other radio button is unselected: false.
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Example 2
We can get the tag name of a radio button using the getTagName() method.
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class RadioButtonTagNames { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will click radio button driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // identify radio button then click WebElement radiobtn = driver.findElement (By.xpath("/html/body/main/div/div/div[2]/form/div[1]/input")); String text = radiobtn.getTagName(); System.out.println("Get the radio button tagname: " + text); // Closing browser driver.quit(); } }
Output
Get the radio button tagname: input Process finished with exit code 0
In the above example, we had obtained the tagname of the radio button in the console with the message - Get the radio button tagname: input.
Example 3
Let us take another example in the below image, where we would get the height, width, x, and y coordinates of the text Selenium - Automation Practice Form on the page using the getRect() method.

Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Rectangle; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ElementPositions { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/radio-button.php"); // Identify the element with xpath locator WebElement e = driver.findElement(By.xpath("/html/body/div/header/div[2]/h1")); Rectangle res = e.getRect(); // Getting height, width, x, and y coordinates of element System.out.println("Getting height: " + res.getHeight()); System.out.println("Getting width: " + res.getWidth()); System.out.println("Getting x-cordinates: " + res.getX()); System.out.println("Getting y-cordinates: " + res.getY()); // Closing browser driver.quit(); } }
Output
Getting height: 29 Getting width: 395 Getting x-cordinates: 453 Getting y-cordinates: 18 Process finished with exit code 0
In the above example, we had obtained the height, width, x and y coordinates of the text in the console with the message - Getting height: 29, Getting width: 395, Getting x-cordinates: 453, and Getting y-cordinates: 18.
Example 4
Let us take another example in the below image, where we would background color the highlighted element Login (depicted in Styles section). We would take the help of the getCssValue() method and pass background-color as a parameter to that method.
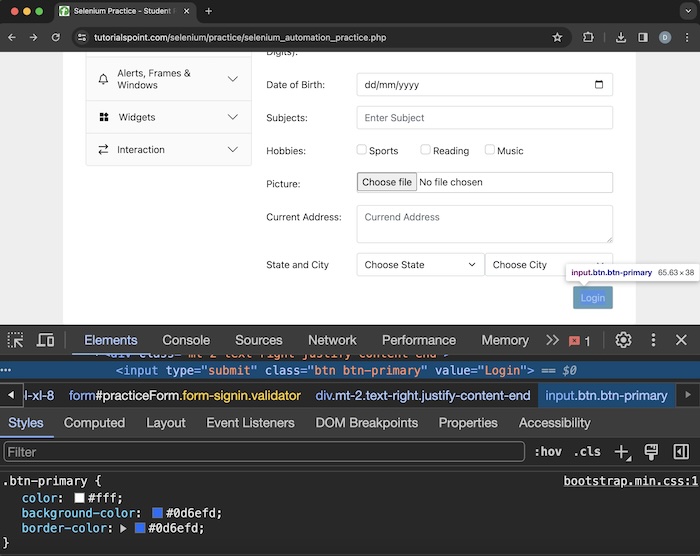
Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ElementsCSSProperty { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the element with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='practiceForm']/div[11]/input")); // Getting background color of the element System.out.println ("Getting background color of element: " + e.getCssValue("background-color")); // Closing browser driver.quit(); } }
Output
Getting background color of element: rgba(13, 110, 253, 1) Process finished with exit code 0
In the above example, we had obtained the background of an element on the page in rgb format in the console with the message - Getting background color of element: rgba(13, 110, 253, 1).
Example 5
Let us take an example of the below page, where we would first enter the text Selenium in the input box with the help of the sendKeys() method. Then we would fetch the runtime value of the element associated with DOM using the getAttribute() method and pass value as a parameter.

Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class InputBoxs { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the element with xpath locator WebElement em = driver.findElement (By.xpath("//*[@id='name']")); // enter text in input box em.sendKeys("Selenium"); // Get the value entered String t = em.getAttribute("value"); System.out.println("Text entered: " + t); // clear the text entered em.clear(); // Get no text after clearing text String t1 = e.getAttribute("value"); System.out.println("Get text after clearing: " + t1); // Closing browser driver.quit(); } }
Output
Entered text is: Selenium Get text after clearing: Process finished with exit code 0
In the above example, we had first entered the text Selenium in the input box, and also retrieved the value entered in the console with the message- Text entered: Selenium. Then cleared the value entered and there was no value in the input box. Hence, we had also received the message in the console: Get text after clearing:.
Example 6
Let us take an example of the below page, where we would obtain the text of the highlighted element - Selenium - Automation Practice Form with the help of the getText() method.

Code Implementation
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class ElementsText { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://www.tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the element with xpath locator WebElement e = driver.findElement (By.xpath("/html/body/div/header/div[2]/h1")); // Getting text of the element System.out.println ("Getting element text: " + e.getText()); // Closing browser driver.quit(); } }
Output
Getting element text: Selenium - Automation Practice Form Process finished with exit code 0
In the above example, we obtained the text of an element in the console with the message - Getting element text: Selenium - Automation Practice Form.
Conclusion
This concludes our comprehensive take on the tutorial on Selenium WebDriver WebElement Commands. Weve started with describing identify radio buttons in HTML, basic web element commands in Selenium Webdriver, and examples to illustrate how to use them in Selenium Webdriver. This equips you with in-depth knowledge of the WebDriver WebElement Commands. It is wise to keep practicing what youve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.